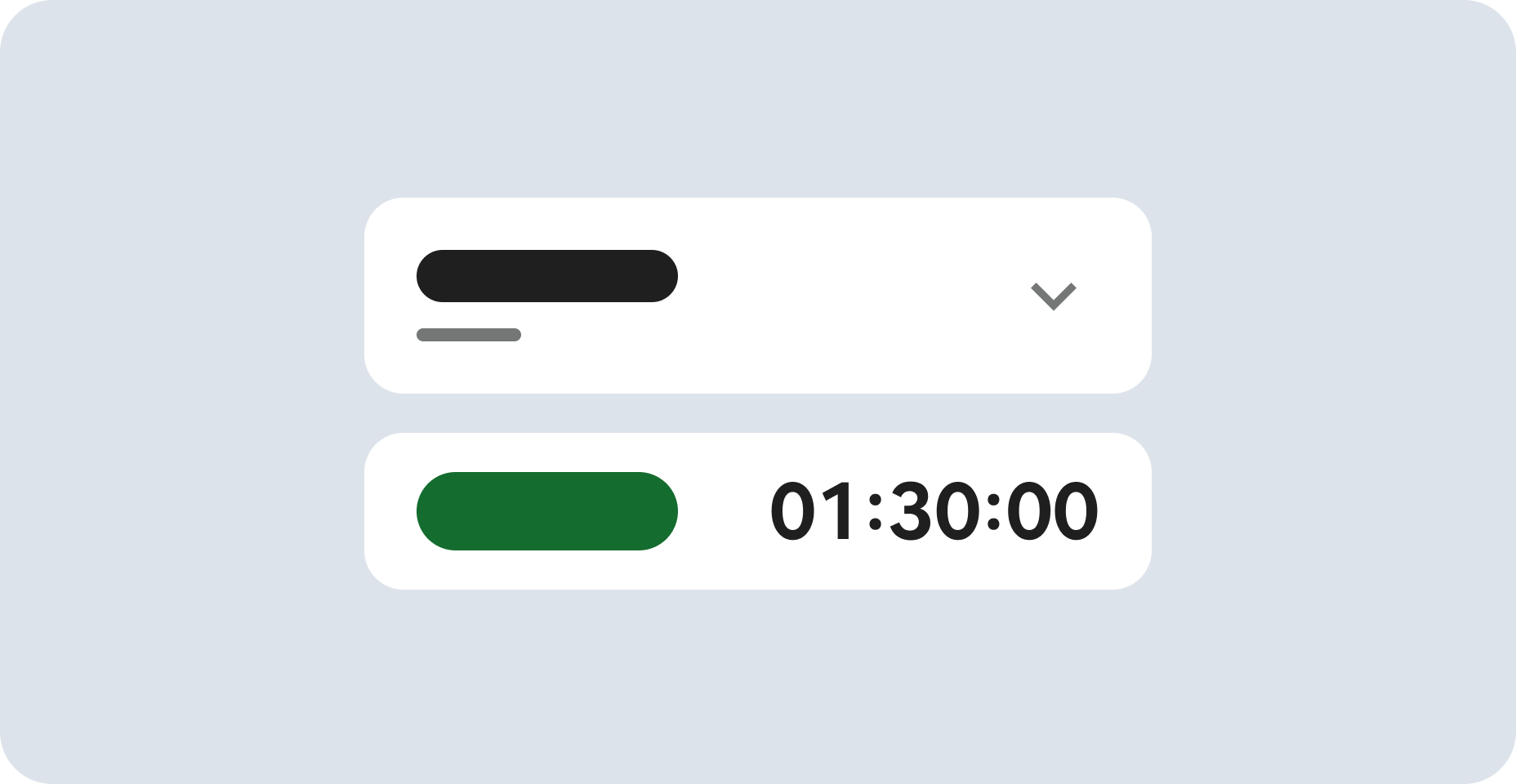
Before you begin
- Labs create a Google Cloud project and resources for a fixed time
- Labs have a time limit and no pause feature. If you end the lab, you'll have to restart from the beginning.
- On the top left of your screen, click Start lab to begin
Lab Completion
/ 10
Dart is a client-optimized language for developing fast apps on any platform. Its goal is to offer the most productive programming language for multi-platform development, paired with a flexible execution runtime platform for app frameworks.
Languages are defined by their technical envelope — the choices made during development that shape the capabilities and strengths of a language. Dart is designed for a technical envelope that is particularly suited to client development, prioritizing both development (sub-second stateful hot reload) and high-quality production experiences across a wide variety of compilation targets (web, mobile, and desktop).
Dart also forms the foundation of Flutter. Dart provides the language and runtimes that power Flutter apps, but Dart also supports many core developer tasks like formatting, analyzing, and testing code.
In this lab, you will learn the basics of Dart in a prepared development environment.
Based on the content, it is recommended to have some familiarity with:
General programming principles
Read these instructions. Labs are timed and you cannot pause them. The timer, which starts when you click Start Lab, shows how long Google Cloud resources are made available to you.
This hands-on lab lets you do the lab activities in a real cloud environment, not in a simulation or demo environment. It does so by giving you new, temporary credentials you use to sign in and access Google Cloud for the duration of the lab.
To complete this lab, you need:
The lab environment includes an Editor and Browser pre-configured for Dart. Access these resources using the lab credentials panel.
IDE
link and paste it into a new browser.In the code editor clone the flutter repository and then access the relevant code sample.
Source Control
.Select Clone Repository
.
Enter the following repository:
With the Repository now cloned onto your environment, the Flutter Workshop repository is now available.
For this lab use the dart folder to complete the exercises.
dart
folder.A solution folder containing working examples is also available in the same repository.
Solution Directory | Contents |
---|---|
dart/lab01/solutions | Variables |
dart/lab02/solutions | Flow control |
dart/lab03/solutions | Functions |
In addition, the following guidelines on Effective Dart will be useful.
To get started with Dart, write a traditional Hello World program and create an application based on the traditional Hello World code.
Create new file hello-world.dart
.
Add the following code:
The program output will be displayed in the debug console.
In this section learn how to declare different types of variables with Dart.
Learn about the following variables types and their use in Dart:
Create an application to use Integers.
hello-integer.dart
.The program output will be displayed in the debug console.
Create an application to use Doubles.
hello-double.dart
.The program output will be displayed in the debug console.
Create an application to use Strings.
hello-string.dart
.The program output will be displayed in the debug console.
Create an application to use Booleans.
hello-bool.dart
.The program output will be displayed in the debug console.
In this section learn how to apply conditional logic in Dart.
Create an IF statement.
hello-if.dart
.The program output will be displayed in the debug console.
Create an IF/ELSE statement.
hello-else.dart
.The program output will be displayed in the debug console.
In this section learn how to declare functions and use them in Dart.
At a high level ypu need to know about the following:
Create an application to use functions without parameters.
hello-function.dart
.The program output will be displayed in the debug console.
Create an application to use functions with parameters.
hello-function2.dart
.The program output will be displayed in the debug console.
Awesome work getting started with Dart.
Click Check my progress to verify the objective.
You have successfully completed the lab and demonstrated your knowledge of Dart. Over the course of this lab, you have been introduced to the following Dart fundamentals:
Manual Last Updated October 11, 2023
Lab Last Tested October 12, 2023
Copyright 2025 Google LLC. All rights reserved. Google and the Google logo are trademarks of Google LLC. All other company and product names may be trademarks of the respective companies with which they are associated.
This content is not currently available
We will notify you via email when it becomes available
Great!
We will contact you via email if it becomes available
One lab at a time
Confirm to end all existing labs and start this one